A developer’s guide to TON APIs and SDKs
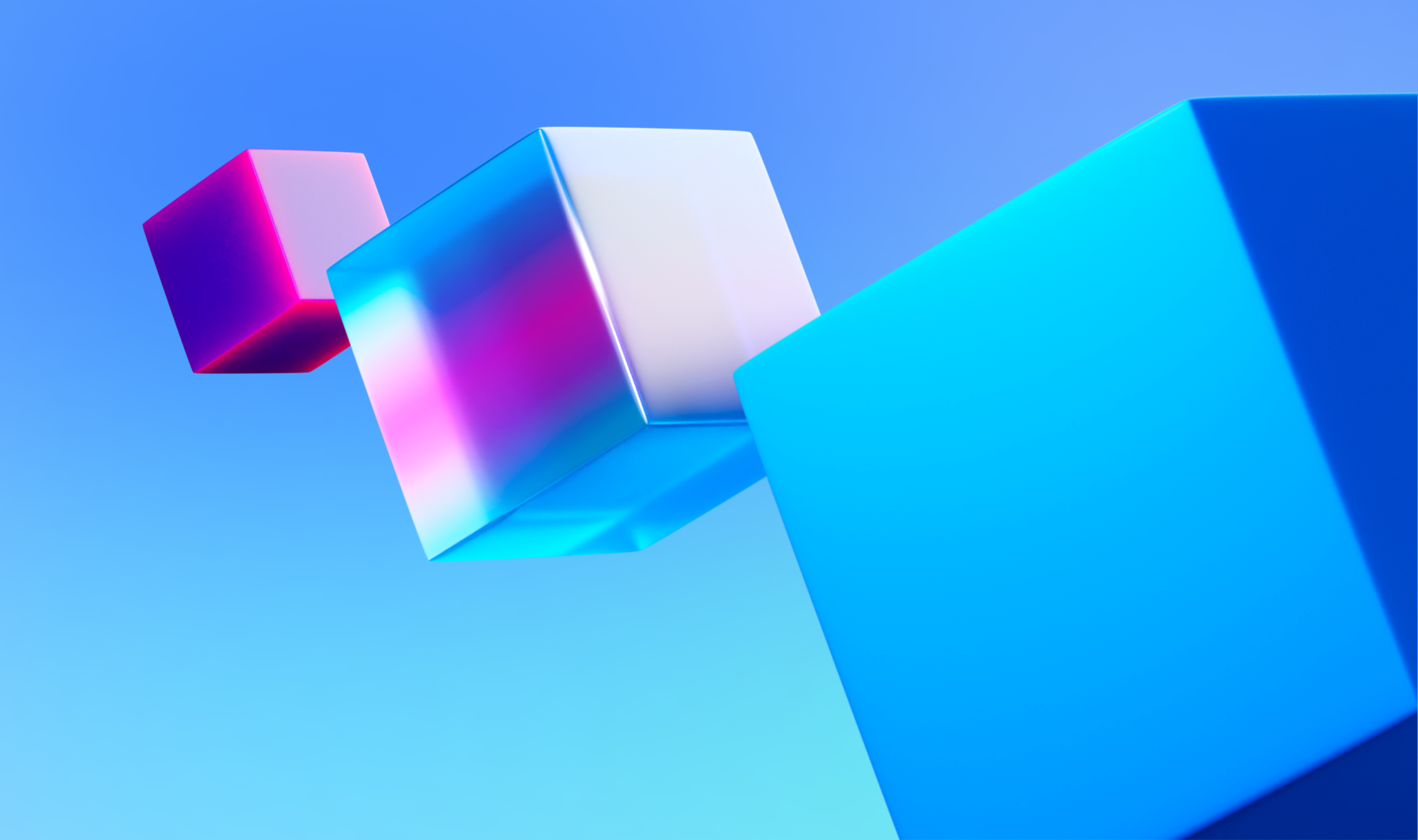
TON Blockchain has increasingly become a magnet for blockchain developers globally, offering a welcoming space for Web3 development. To support this growth, we’ve put together a range of tools and libraries to simplify the process of building on TON Blockchain.
Whether you’re looking to write code quickly (@ton/blueprint
), interact with blockchain systems (@ton/ton, @ton-community/assets-sdk, @tonconnect/sdk
), or build and test applications (@ton/core, @ton/crypto, @ton/sandbox)
, we’ve got you covered.
In the coming sections, we'll explain each library and show you how to use them with simple code examples.
@ton/ton
@ton/ton
@ton/ton is a popular JavaScript/TypeScript library designed to interact with TON Blockchain. It allows developers to fetch wallet and blockchain data and execute transactions.
Here are a few examples:
Creating a TON client
A TON client is an abstraction layer for interacting with the blockchain. You can set the endpoint to either the testnet or mainnet.
const tonClient = new TonClient({
endpoint: 'https://testnet.toncenter.com/api/v2/jsonRPC'
});
Creating a wallet
function createWallet() {
let wallet = WalletContractV4.create({ publicKey: Buffer.from('[YOUR_PUBLIC_KEY]'), workchain: 0 });
return tonClient.open(wallet);
}
Creating a wallet balance
const wallet = createWallet();
let balance = await wallet.getBalance();
Sending a transaction
const wallet = createWallet();
let seqno = await wallet.getSeqno();
await wallet.sendTransfer({
seqno,
secretKey: Buffer.from('[YOUR_SECRET_KEY]'),
messages: [internal({
value: 100000n,
to: '[ADDRESS]'
})]
});
In addition to standard wallets, the library supports other contracts, including JettonMaster, JettonWallet, MultisigOrder, MultisigWallet,
and ElectorContract
. It also includes functions for parsing configs, calculating gas fees, and more.
@ton-community/assets-sdk
@ton-community/assets-sdk
The @ton-community/assets-sdk is the easiest way to manage assets on TON. It provides a developer-friendly API for interacting with unique assets, including NFTs, SBTs, and Jettons.
The SDK includes pre-built solutions for Jetton and NFT contracts. You can use these as templates or starting points for creating custom wrappers, streamlining development and accelerating integration.
To start using this SDK, you need to set up an instance of AssetsSDK
in your code. This will involve:
- Specifying a storage option: Currently, Pinata and S3 are supported out of the box, but you can implement your own storage solution via the interface.
- Selecting an API: Choose between mainnet and testnet.
- Configuring a wallet: V4 is supported out of the box.
Here’s a quick example to get you started:
import {AssetsSDK, PinataStorageParams, createApi, createSender, createWalletV4, importKey
} from "@ton-community/assets-sdk";
// create an instance of the TonClient4
const NETWORK = 'testnet';
const api = await createApi(NETWORK);
// create a sender from the wallet (in this case, Highload Wallet V2)
const keyPair = await importKey(process.env.MNEMONIC);
const sender = await createSender('highload-v2', keyPair, api);
// define the storage parameters (in this case, Pinata)
const storage: PinataStorageParams = {
pinataApiKey: process.env.PINATA_API_KEY!,
pinataSecretKey: process.env.PINATA_SECRET!,
}
// create the SDK instance
const sdk = AssetsSDK.create({
api: api, // required, the TonClient4 instance
storage: storage, // optional, the storage instance (Pinata, S3 or your own)
sender: sender, // optional, the sender instance (WalletV4, TonConnect or your own)
});
Here, you’ll need to pass your Pinata keys and the wallet mnemonic (space separated) as environment variables.
Note: Don't use the mnemonic in the browser environment. Opt for TonConnect instead.
Once everything is set up, you can:
- To create a Jetton minter or an NFT/SBT collection, use the methods
createJetton
orcreateNftCollection/createSbtCollection
. - Open an existing Jetton minter, Jetton wallet, NFT/SBT collection, or NFT/SBT item to perform actions such as minting, transferring, or burning.
@ton/blueprint
@ton/blueprint is an environment for developing, testing, and deploying smart contracts. With various commands, it allows developers to build contracts, run scripts, and execute tests.
For example, to build a contract, you can use the following command:
npx blueprint build <contract>
This command compiles the contract located at contracts/<contract>.
To run a script, you need to use the command below:
npx blueprint run <script>
This command runs the script located at scripts/<script>.
Some other commands include:
- **
npx blueprint create:** to create a contract
npx blueprint test: to run tests
Here's an example of a deployment script:
import { toNano } from '@ton/core';
import { Test } from '../wrappers/Test';
import { compile, NetworkProvider } from '@ton/blueprint';
export async function run(provider: NetworkProvider) {
const test = provider.open(
Test.createFromConfig(
{
id: Math.floor(Math.random() * 10000),
counter: 0,
},
await compile('Test')
)
);
await test.sendDeploy(provider.sender(), toNano('0.05'));
await provider.waitForDeploy(test.address);
}
@ton/sandbox
@ton/sandbox lets you emulate the blockchain, create smart contracts, send messages, view logs, and use GET methods.
To start emulating, you need to create a blockchain instance.
import { Blockchain } from "@ton/sandbox";
const blockchain = await Blockchain.create()
This sets up a local blockchain environment for testing and development.
Once your blockchain instance is set up, you can use the low-level methods on blockchain, such as sendMessage
, to emulate any messages you need. However, it’s recommended to write wrappers for your contract using the Contract interface from @ton/core. You can then use blockchain.openContract
on those contract instances. This wraps them in a Proxy that supplies a ContractProvider
as the first argument to any method that starts with get or send.
In addition, all send methods will be promisified. This means that they will return the method’s result in the result field, along with the outcomes from the blockchain message queue.
@ton/core
@ton/core is the main library for developing on TON. It provides essential tools for serializing and deserializing almost everything you’ll encounter on TON Blockchain.
Here’s an example for assembling a message:
const message = beginCell()
.storeUint(0x1, 32)
.storeCoins(123321)
.storeAddress(Address.parse(‘YOUR_ADDRESS’))
.storeMaybeRef(beginCell()
.storeBit(1)
.endCell()
)
.storeStringTail('String')
.endCell();
Here’s an example of deserializing this message:
const sc = message.beginParse();
const op = sc.loadUint(32);
const coins = sc.loadCoins();
const addr = sc.loadAddress();
const maybeRef = sc.loadMaybeRef();
let bit: boolean | null = null;
if (maybeRef) {
const sc = maybeRef.beginParse();
bit = sc.loadBit();
}
const str = sc.loadStringTail();
In addition to serializing and deserializing structures, messages, and tuples, @ton/core
includes key features such as Address, Dictionary, and exotic cells. It also provides a Sender interface for sending messages and allows for the creation of Merkle proofs.
Overall, @ton/core
is your go-to tool for developing on TON.
@ton/crypto
@ton/crypto is a cryptographic library essential for secure interactions on TON Blockchain. It supports working with mnemonics and cryptographically secure random numbers.
Here’s how to generate a mnemonic and a pair of private and public keys:
const mnemonics = await mnemonicNew(24); // Generate new mnemonics
const keypair: KeyPair = await mnemonicToPrivateKey(mnemonics);
@tonconnect/sdk
@tonconnect/sdk is a comprehensive communication protocol for seamlessly integrating TON wallets. It comprises the following set of packages:
@tonconnect/sdk allows you to connect your app to TON wallets via the TonConnect protocol. To do this, you’ll need to call restoreConnection.
The connection will be restored if the user has previously connected their wallet.
import TonConnect from '@tonconnect/sdk';
const connector = new TonConnect({
manifestUrl: 'https://myApp.com/assets/tonconnect-manifest.json'
});
connector.restoreConnection();
@tonconnect/protocol includes protocol requests, responses, event models, and encoding and decoding functions.
Here’s how to use protocol models in your app:
import { AppRequest, RpcMethod, WalletResponse } from '@tonconnect/protocol';
function myWalletAppRequestsHandler<T extends RpcMethod>(request: AppRequest<T>): Promise<WalletResponse<T>> {
// handle request, ask the user for a confirmation and return WalletResponse
}
Here’s how to use protocol cryptography in your app:
import { SessionCrypto, WalletMessage, Base64, hexToByteArray }
from '@tonconnect/protocol';
function encodeIncommingHTTPBridgeRequest(encryptedMessage: string, from: string): WalletMessage {
const sessionCrypto = new SessionCrypto(yourStoredSessionReypair);
const decryptedMessage =
sessionCrypto.decrypt(
Base64.decode(bridgeIncomingMessage.message).toUint8Array(),
hexToByteArray(bridgeIncomingMessage.from)
);
return JSON.parse(decryptedMessage);
}
@tonconnect/ui is a UI kit for the TonConnect
SDK.
Here’s how to create a TonConnectUI
instance:
import TonConnectUI
from '@tonconnect/ui'
const tonConnectUI = new TonConnectUI({
manifestUrl: 'https://<YOUR_APP_URL>/tonconnect-manifest.json',
buttonRootId: '<YOUR_CONNECT_BUTTON_ANCHOR_ID>'
});
Calling this method await tonConnectUI.openModal();
will display the modal window and return a promise that resolves after the modal window is opened.
@tonconnect/ui-react is a React UI kit for the TonConnect SDK. Make sure to place all TonConnect UI hooks calls and the <TonConnectButton
/> component inside <TonConnectUIProvider>.
import { TonConnectUIProvider }
from '@tonconnect/ui-react';
export function App() {
return (
<TonConnectUIProvider manifestUrl="https://<YOUR_APP_URL>/tonconnect-manifest.json">
{ /* Your app */ }
</TonConnectUIProvider>
);
}
Use the TonConnectModal
hook to access the functions for opening and closing the modal window. The hook returns an object with the current modal state and methods to open and close the modal.
import { useTonConnectModal }
from '@tonconnect/ui-react';
export const ModalControl = () => {
const { state, open, close } = useTonConnectModal();
return (
<div>
<div>Modal state: {state?.status}</div>
<button onClick={open}>Open modal</button>
<button onClick={close}>Close modal</button>
</div>
);
};
Use the TonWallet hook to retrieve the currently connected TON wallet. If no wallet is connected, the hook will return null.
import { useTonWallet }
from '@tonconnect/ui-react';
export const Wallet = () => {
const wallet = useTonWallet();
return (
wallet && (
<div>
<span>Connected wallet: {wallet.name}</span>
<span>Device: {wallet.device.appName}</span>
</div>
)
);
};
Summing up
Every great technological leap begins with a single line of code and the determination to build something meaningful. The tools and libraries available provide the building blocks to create impactful solutions, from smart contracts to asset management.
The true potential lies in how you, the developer, take these tools and use them to influence the blockchain ecosystem. Your journey evolves with each experiment, every interaction, and all the knowledge you integrate into your projects. Dive deeper, collaborate with the community, and explore the ever-expanding ecosystem of TON.